123123123
Erfahrenes Mitglied
Hallo!
Seit kurzem programmiere ich eine App für Android. Die App wird ein Cocktailmixer!
Dabei habe ich mit SQLiteOpenHelper Datenbanken erstellt.
So sieht die im Ganzen aus. In der Tabelle Zutaten habe ich schon mal paar Zutaten eingefügt:
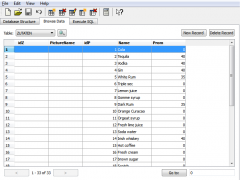
Ich hab mir so gedacht, wenn man auf Button Zutaten klickt, kommt man auf eine neue Seite und da sollen die Zutaten untereinander stehen
Jetzt meine Frage: Wie kriege ich diese alle Zutaten ausgegeben? Am besten tabellarisch.
Codes:
MainActivity.java
SecondMainActivity.java (wenn man Zutaten klickt, kommt man auf diese Seite)
DataBaseManager.java (Die Datenbank)
Würde mich sehr über eine Antwort freuen.
Danke!

Seit kurzem programmiere ich eine App für Android. Die App wird ein Cocktailmixer!
Dabei habe ich mit SQLiteOpenHelper Datenbanken erstellt.
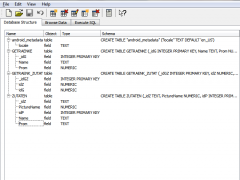
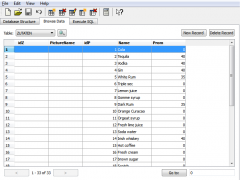
Ich hab mir so gedacht, wenn man auf Button Zutaten klickt, kommt man auf eine neue Seite und da sollen die Zutaten untereinander stehen
Jetzt meine Frage: Wie kriege ich diese alle Zutaten ausgegeben? Am besten tabellarisch.
Codes:
MainActivity.java
Code:
package com......;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
public class MainActivity extends Activity implements OnClickListener {
//testButtons
private Button BtnZutaten, BtnPromZutaten, BtnGetraenke;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//testButtons
BtnZutaten = (Button)findViewById(R.id.btnZutaten);
BtnPromZutaten = (Button)findViewById(R.id.BtnPromZutaten);
BtnGetraenke = (Button)findViewById(R.id.BtnGetraenke);
BtnZutaten.setOnClickListener(this);
BtnPromZutaten.setOnClickListener(this);
BtnGetraenke.setOnClickListener(this);
//auf andere Seite zu kommen
Button BtnZutaten = (Button)findViewById(R.id.btnZutaten);
BtnZutaten.setOnClickListener(new View.OnClickListener()
{
public void onClick(View v) {
Intent in = new Intent
(MainActivity.this,SecondMainActivity.class);
startActivity(in);
}
});
}
//onClick from specific testButton
public void onClick(View v){
switch(v.getId()){
case R.id.btnZutaten:
alleZutaten();
break;
case R.id.BtnPromZutaten:
allePromille();
break;
case R.id.BtnGetraenke:
alleGetraenke();
break;
}
}
//method
public void alleZutaten(){
}
public void allePromille(){
}
public void alleGetraenke(){
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
SecondMainActivity.java (wenn man Zutaten klickt, kommt man auf diese Seite)
Code:
package com.......;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
public class SecondMainActivity extends Activity implements OnClickListener {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.list);
}
public void onClick(View v) {
}
}
DataBaseManager.java (Die Datenbank)
Code:
package com..........;
import android.database.Cursor;
import android.database.SQLException;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteException;
import android.database.sqlite.SQLiteOpenHelper;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.ArrayList;
public class DataBaseManager extends SQLiteOpenHelper {
// The Android's default system path of the application database.
//data/data/ and /databases remain the same always. The one that must be changed is com.example which represents
//the MAIN package of the project
private static String DB_PATH = "/data/data/com.masters.cocktailmixer/databases/";
//the name of the database
private static String DB_NAME = "cocktaildb";
private static SQLiteDatabase mDataBase;
private static DataBaseManager sInstance = null;
// database version
private static final int DATABASE_VERSION = 1;
//name of database tables and columns
private static final String TABLE_ZUTATEN = "ZUTATEN";
private static final String TABLE_GETRAENKE = "GETRAENKE";
private static final String TABLE_GETRAENK_ZUTAT = "GETRAENK_ZUTAT";
private static final String COLUMN_NAME = "Name";
private static final String COLUMN_PROM = "Prom";
private static final String COLUMN_PICTURE = "PictureName";
/**
* Constructor Takes and keeps a reference of the passed context in order to
* access to the application assets and resources.
*/
private DataBaseManager() {
super(ApplicationContextProvider.getContext(), DB_NAME, null, DATABASE_VERSION);
try {
createDataBase();
openDataBase();
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* Singleton for DataBase
*
* @return singleton instance
*/
public static DataBaseManager instance() {
if (sInstance == null) {
sInstance = new DataBaseManager();
}
return sInstance;
}
/**
* Creates a empty database on the system and rewrites it with the own
* database.
*
* @throws java.io.IOException io exception
*/
private void createDataBase() throws IOException {
boolean dbExist = checkDataBase();
if (dbExist) {
// do nothing - database already exist
} else {
// By calling this method an empty database will be created into
// the default system path
// of the application so we are gonna be able to overwrite that
// database with our database.
this.getReadableDatabase();
try {
copyDataBase();
} catch (IOException e) {
throw new Error("Error copying database");
}
}
}
/**
* Check if the database already exist to avoid re-copying the file each
* time you open the application.
*
* @return true if it exists, false if it doesn't
*/
private boolean checkDataBase() {
SQLiteDatabase checkDB = null;
try {
String myPath = DB_PATH + DB_NAME;
checkDB = SQLiteDatabase.openDatabase(myPath, null,
SQLiteDatabase.OPEN_READONLY);
} catch (SQLiteException e) {
// database doesn't exist yet.
}
if (checkDB != null) {
checkDB.close();
}
return checkDB != null;
}
/**
* Copies the database from the local assets-folder to the just created
* empty database in the system folder, from where it can be accessed and
* handled. This is done by transfering bytestream.
*
* @throws java.io.IOException io exception
*/
public void copyDataBase() throws IOException {
// Open the local db as the input stream
InputStream myInput = ApplicationContextProvider.getContext().getAssets().open(DB_NAME);
// Path to the just created empty db
String outFileName = DB_PATH + DB_NAME;
// Open the empty db as the output stream
OutputStream myOutput = new FileOutputStream(outFileName);
// transfer bytes from the inputfile to the outputfile
byte[] buffer = new byte[1024];
int length;
while ((length = myInput.read(buffer)) > 0) {
myOutput.write(buffer, 0, length);
}
// Close the streams
myOutput.flush();
myOutput.close();
myInput.close();
}
private void openDataBase() throws SQLException {
// Open the database
String myPath = DB_PATH + DB_NAME;
mDataBase = SQLiteDatabase.openDatabase(myPath, null,
SQLiteDatabase.OPEN_READWRITE);
}
/**
* Select method
*
* @param query select query
* @return - Cursor with the results
* @throws android.database.SQLException sql exception
*/
public Cursor select(String query) throws SQLException {
return mDataBase.rawQuery(query, null);
}
/**
* Let you make a raw query
*
* @param command - the sql comand you want to run
*/
public void sqlCommand(String command) {
mDataBase.execSQL(command);
}
/**
* Let you select the content from the specific table
* The data gets 'converted' from an ArrayList to a StringArray
* Returns the result
**/
public String[] getIngredients(){
Cursor c = mDataBase.rawQuery("SELECT * FROM " + TABLE_ZUTATEN,null);
ArrayList<String> list = new ArrayList<String>();
if (c.moveToFirst())
{
do
{
String s = c.getString(c.getColumnIndex(COLUMN_NAME));
list.add(s);
}
while (c.moveToNext());
}
if (c != null && !c.isClosed())
{
c.close();
}
String[] result = new String[list.size()];
result = list.toArray(result);
return result;
}
/**
* Let you select the content from the specific table
* Returns the result
**/
public String getAlcoholicStrength(Object checkedItem){
String result = null;
Cursor c = mDataBase.rawQuery("SELECT " + COLUMN_PROM + " FROM " + TABLE_ZUTATEN + " WHERE " + COLUMN_NAME + " = '" + checkedItem.toString()+"'",null);
if (c.moveToFirst())
{
do
{
result = c.getString(c.getColumnIndex(COLUMN_PROM));
}
while (c.moveToNext());
}
if (c != null && !c.isClosed())
{
c.close();
}
return result;
}
/**
* Let you select the content from the specific table
* The data gets 'converted' from an ArrayList to a StringArray
* Returns the result
**/
public String[] getDrinks(){
ArrayList<String> list = new ArrayList<String>();
Cursor c = mDataBase.rawQuery("SELECT * FROM " + TABLE_GETRAENKE,null);
if (c.moveToFirst())
{
do
{
String s = c.getString(c.getColumnIndex(COLUMN_NAME));
list.add(s);
}
while (c.moveToNext());
}
if (c != null && !c.isClosed())
{
c.close();
}
String[] result = new String[list.size()];
result = list.toArray(result);
return result;
}
public String[] getPicture(){
ArrayList<String> list = new ArrayList<String>();
Cursor c = mDataBase.rawQuery("SELECT * FROM " + TABLE_GETRAENK_ZUTAT,null);
if (c.moveToFirst())
{
do
{
String s = c.getString(c.getColumnIndex(COLUMN_PICTURE));
list.add(s);
}
while (c.moveToNext());
}
if (c != null && !c.isClosed())
{
c.close();
}
String[] result = new String[list.size()];
result = list.toArray(result);
return result;
}
@Override
public synchronized void close() {
if (mDataBase != null)
mDataBase.close();
super.close();
}
@Override
public void onCreate(SQLiteDatabase db) {
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
}
}
Würde mich sehr über eine Antwort freuen.
Danke!

